I looked around online and couldn’t find a good documentation about using Highcharts in Vue with data coming from a database. This solution worked for me, but feel free to comment suggestions or better implementations!
I also assume you have a working Vue website, and basic understanding of Vue and javascript. lol
First you will need to import Axios and highcharts
https://www.npmjs.com/package/axios
https://www.npmjs.com/package/highcharts-vue
npm i highcharts-vue
npm i axios
Next, we can import these packages to our component
import axios from "axios";
import {Chart} from 'highcharts-vue'
I would like to walk you through the rest of this line by line, but most of you will appreciate all the rest of the code.
export default {
name: 'yourComponentName',
props: {
msg: String
},
components: {
highcharts: Chart
},
data() {
return {
chartOptions: {
chart: {
style: {
color: "white"
}
},
tooltip: {
style: {
color: 'white',
fontWeight: 'bold'
}
},
title: {
text: 'Current year profit',
style: {
color: 'white'
}
},
xAxis: {
type: 'category',
labels: {
rotation: -45,
style: {
fontSize: '13px',
fontFamily: 'Verdana, sans-serif',
color: 'white'
}
}
},
yAxis: {
min: 0,
title: {
text: 'Dollars',
style:{
color:'white'
}
}
},
series: [{
data: null,
name: "Sum of profit by month"
}],
legend: {
itemStyle: {
color: 'white',
fontWeight: 'bold'
}
}
}
}
},
mounted() {
axios.get("http://yourAPI.com/GetData")
.then(response => {
var series_data = [], thedata= response.data;
for (var i=0; i< thedata.length; i++) {
series_data.push([thedata[i].month_, thedata[i].sum])
}
this.chartOptions.series[0].data = series_data
}).catch(err => {
})
}
}
Essentially, the key here is setting up your chart options. Then when you access your component, the mounted HTTP call with axios will fill in your chart series data. If you need further explanation, please comment and I will edit this post to add more information.
Last, call your highcharts object with the options you set up in the template.
<template>
<div>
<highcharts :options="chartOptions"></highcharts>
</div>
</template>
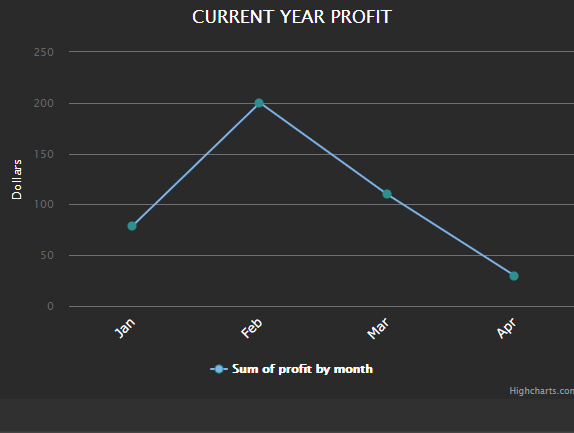
I enjoy, cause I discovered exactly what I was having a look for. You’ve ended my four day long hunt! God Bless you man. Have a nice day. Bye
Savedd ass a favorite, I reallly lime your blog!