I needed to change a single select dropdown to a multi select. It was easier than I thought, essentially it was a one liner. Here is the code to setup a simple dropdown and multi-select. I assume you have a basic understanding of C# MVC Razor pages.
First lets set up the model
public class myClass
{
public List<dropdown> myDropdown {get;set;}
public string dropdownItem {get;set;}
public myClass()
{
}
public class dropdown
{
public int ID {get;set;}
public string NAME {get;set;}
}
public static List<dropdown> FILL_DROPDOWN()
{
//your logic to fill in dropdown
//I use dapper so my logic will get from a db, something like this
// var dropdown = conn.Query<dropdown>(sql).ToList();
//return dropdown
}
}
Next lets do our controller.
public class HomeController : Controller
{
public ActionResult Index()
{
myClass model = new myClass();
model.myDropdown = myClass.FILL_DROPDOWN();
return View(model);
}
}
Now we can add our dropdowns to the razor page.
@model APP.Models.myClass //or wherever your model is
<div>
@Html.ListBoxFor(m => m.dropdownItem, new SelectList(Model.myDropdown, "ID", "NAME"), new { id = "yourID", @class = "form-control", style = "height:200px" })
@Html.DropDownListFor(m => m.dropdownItem , new SelectList(Model.myDropdown, "ID", "NAME"), "select...", new { id = "yourID", @class = "form-control" })
</div>
the DropDownListFor (single select dropdown) has a lot of overloads, in the example above, I’m passing the selection, the selectList of items, an optionLabel (the “select…”), and the htmlAttributes (class, id, styles, etc).
similarly for the the ListBoxFor (multi select), in the example above, I’m passing the selection, the selectList of items, and the htmlAttributes (class, id, styles, etc).
Below is our rendered multi select and single select objects. I am using bootstrap class “form-control” so my dropdown may look different from yours.
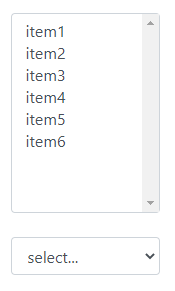
Pretty simple stuff. just changing one word changes from a single select dropdown to a list. This Razor markup is really customizable too, check out the other overloads and mess around.